I recently took on a skills assessment test for a job that required building out pages using bootstrap and sass. The challenge was deceivingly simple but, like any project, it didn’t take long for the stylesheet to get crowded and harder to manage. As I always say, writing code is like solving a puzzle that you create yourself in real-time.
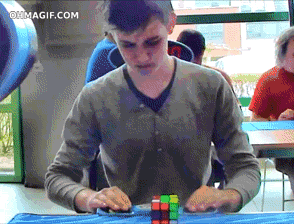
In an attempt to make the stylesheet more manageable and easier to edit, I began using mixins for media queries. I also added in variables in place of screen sizes so that they can be changed in a single area of the document later. The variables along with the mixin looked something like this:
$screen-lg: 1200px;
@mixin screen-size($size) {
@media (min-width: $size) {
@content;
}
}
By creating a set of variables, I can now use @include whenever I needed to use them. Like this:
div {
//Some style here
@include screen-size($screen-lg) {
//New styles here
}
}
I like this method a lot because not only is it making for an elegant solution for de-cluttering the css, it’s also really simple to reuse over and over and it makes it so that you don’t have to hunt down where that media query is for that particular class name. This works even better if you have multiple breakpoints too, like this:
$screen-lg: 1200px;
$screen-md: 820px;
$screen-sm: 414px;
@mixin screen-size($size) {
@media (min-width: $size) {
@content;
}
}
div {
font-family: Helvetica;
font-weight: normal;
@include screen-size($screen-lg) {
font-size: 3rem;
}
@include screen-size($screen-md) {
font-size: 2rem;
}
@include screen-size($screen-sm) {
font-size: 1rem;
}
}
Here’s what it looks like after it’s been compiled:
div {
font-family: Helvetica;
font-weight: normal;
}
@media screen and (min-width: 1200px) {
div {
font-size: 3rem;
}
}
@media screen and (min-width: 820px) {
div {
font-size: 2rem;
}
}
@media screen and (min-width: 414px) {
div {
font-size: 1rem;
}
}
To learn about other ways you can use mixins https://sass-lang.com/documentation/at-rules/mixin